In Java Wrapper classes are used to treat primitive data types like objects. int becomes Integer and boolean becomes Boolean. But did you know that also the keyword void has a wrapper class? We will look at when and how this is useful.
OBJECT ORIENTED?
If you are not familiar with Wrapper classes in Java already, I try to give a quick explanation. You might know that Java is a Object oriented programming language. What that means is that after creating a class you can use the class as a template for multiple Objects.
Lets say you have a class car which includes a name,brand and a price. Using the new keyword you can create all sorts of cars and access the methods within the object as seen in the picture below
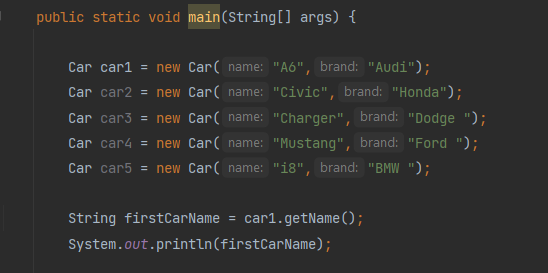
WHAT IS A WRAPPER CLASS
In Java there are two types of data types, primitive and reference data types. The reference data types already reference to an object like an Array, Strings are also reference data types. The 8 primitive data types like int, double and boolean are commonly used in Java. Sometimes you want a data type like int to act like an object, for example if you want a ArrayList of int you need to pass Integer as the data type. Wrapper Classes are used to convert these primitive data types into objects.
So.. what is a Void wrapper class
The void wrapper class has been around for some time now. It was first implemented in java 1.1 the
version between Oak and Playground. It was originally created for java reflection but now it is also
used in the context of generics which I will come to in a moment.
The void wrapper class is not a wrapper class in true essence, since void is not considered a primitive
datatype it is merely a java keyword even doe the eclipse documentation lists void as primitive data
type and the function isPrimitive returns true when called by Void.Type.
This is a debate for another time we won't get into that right now. Just bare in mind that void is not a
primitive data type.

Said so the Void class can not be initialized using the new keyword neither does it store a value of type void which is stated in the Void class itself. The only useful content inside the Void class is the static variable TYPE which references to the getPrimitiveClass method inside the class Class.
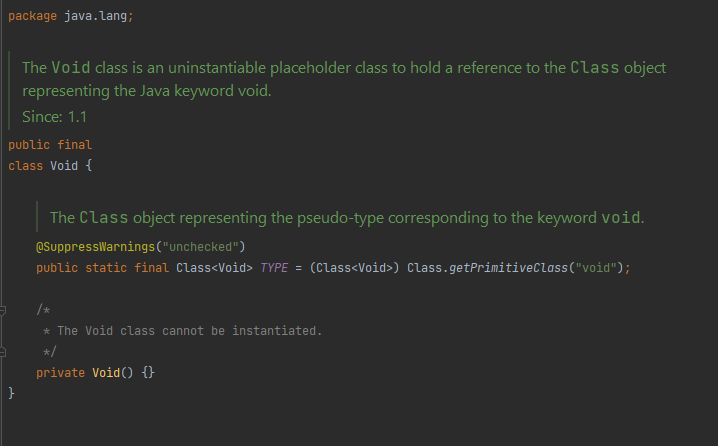
According to the javadoc the void wrapper class exists because some times you may need to represent the
keyword void as an object.
That is kind of confusing because as just said it can not be initialized caused by the constructor in
the Void class being declared private. The Void class is also a final class and because of this none of
the included features can be inherited by another class, it is also not possible to extend a final class
One reasons to use the Wrapper class Void is to set the return type of a method as Void and
still return something different to the keyword void which does not require a return statement at the
end of the function. In the case of return type Void the only valid return value would be null.
A real life example of this is the doPrivileged method in the AccessController class, you can check out
here
generally speaking Void is widely used for callback functions when you need to pass a value that does
not exist.

Generics
This is also where generics come into play. Since java 5 after generics were implemented the void wrapper class can also be used to indicate that classes do not return a value. More broadly what that means is if something requires you to provide a return type but nothing is returned you can use the void wrapper class to indicate that nothing is returned. a good example of this is implementing Callable. Callable are further explained here. Void is also sometimes used in a Map, indicating that the key is not used and the value is returned.

Reflection
Reflection is the ability to inspect the class structure of an object at runtime. Because I am not an
expert on java reflection I will not go into detail here. One implementation of the void wrapper class i
found was in the java reflection API. This api uses Void.TYPE to check the return type of a method.
assert(handleForTest.getReturnType() == Void.TYPE);